1. 配置文件格式
SpringBoot
配置文件格式支持后缀名为.yml
和.properties
两种格式的文件。后缀名为.yml
的文件是通过YAML
语法编写;
@@注意: 如果application.yml
和application.properties
两个文件同时存在相同的路径下,则优先使用application.properties
配置文件的内容。关于配置文件的加载优先级,后面小节详细归纳。
2. 准备测试条件
为了方便验证配置文件中的值是否能被正确读取到,新建立Person
类和Job
类,以备后续使用。最终效果是通过配置文件设置这两个类的属性值。
2.1 创建Job类
新建文件: Job.java
文件位置: src/main/java/com/hui/configfile/bean/Job.java
package com.hui.configfile.bean;
import lombok.Data; import org.springframework.stereotype.Component;
@Data @Component public class Job { private String jobName; private Integer jobAge; @Override public String toString() { return "Job{" + "jobName='" + jobName + '\'' + ", jobAge=" + jobAge + '}'; } }
|
2.2 创建Person类
新建文件: Person.java
文件位置: src/main/java/com/hui/configfile/bean/Person.java
package com.hui.configfile.bean;
import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component;
import java.util.Date; import java.util.List; import java.util.Map; @Data @Component public class Person { private String name; private Integer age; private Boolean isBoy; private Date birthday; private Map<String,Object> other; private List<String> likes; private Job job;
@Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + ", birthday=" + birthday + ", isBoy=" + isBoy + ", other=" + other.toString() + ", likes=" + likes.toString() + ", job =" + job.toString() + '}'; } }
|
2.3 引入配置文件处理器
导入配置文件处理器,配置文件进行绑定就会有提示
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring‐boot‐configuration‐processor</artifactId> <optional>true</optional> </dependency>
|
效果如下:
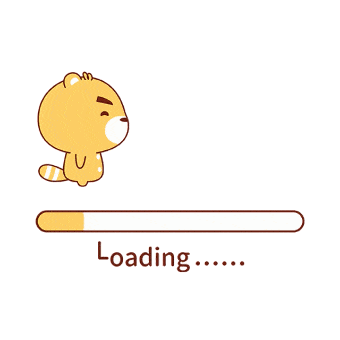
3. 注入到对象(@ConfigurationProperties
)
3.1 使用(application.yml
)
修改文件: application.yml
server: port: 8001 person: name: 张三 age: 25 birthday: 1996/11/23 is-boy: true other: { home: 北京, phone: 17600000000 } likes: - 游戏 - 动漫 - 游泳 job: jobName: 开发工程师 job-age: 3
|
上述配置中is-boy
也可以写成: isBoy
3.1.1 注入对象
为了方便验证Yaml
值的写法和赋值效果,修改Person
对象,使@ConfigurationProperties
用来加载配置文件的内容;
@ConfigurationProperties
:告诉SpringBoot
将本类中的所有属性和配置文件中相关的配置进行绑定,prefix = "person"
:配置文件中哪个下面的所有属性进行一一映射
import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component;
import java.util.List; import java.util.Map; @Data @Component @ConfigurationProperties(prefix = "person") public class Person { .... }
|
3.1.2 测试代码
package com.hui.configfile;
import com.hui.configfile.bean.Person; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest class ConfigFileApplicationTests { @Autowired Person person; @Test void contextLoads() { System.out.println(person); } }
|
3.2 使用(application.properties
)
修改文件:application.properties
server.port=8002
person.name=小美
person.age=21
person.is-boy=false
person.other.home=北京 person.other.phone=110110110
person.likes=游戏,动漫,游泳
person.birthday=2022/05/17
person.job.jobName=模特 person.job.jobAge=2
|
3.2.1 注入并测试
配置绑定到对象代码同(3.1.1 注入对象
) 测试代码同(3.1.2 测试代码
)
输出乱码:
Person{name='å°ç¾', age=21, birthday=Tue May 17 00:00:00 CST 2022, isBoy=false, other={home=å京, phone=110110110}, likes=[游æ, 卿¼«, 游泳], job =Job{jobName='模ç¹', jobAge=2}}
|
properties
配置文件在idea中默认utf-8会乱码,如上输出。可进行以下调整
配置File Encodings
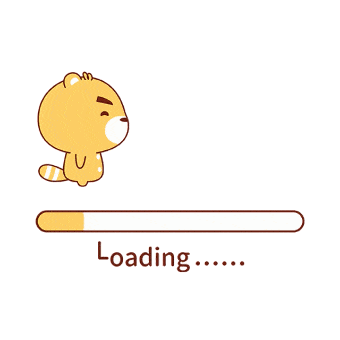
如果IDEA版本是2020的,上面设置以后,控制台输出还是乱码,还需要进行以下配置
设置 Additional command line parameters选项为 -encoding utf-8,如下图:
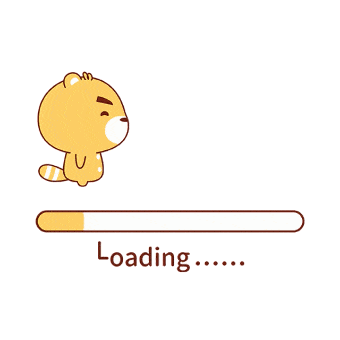
设置后执行输出:
Person{name='小美', age=21, birthday=Tue May 17 00:00:00 CST 2022, isBoy=false, other={home=北京, phone=110110110}, likes=[游戏, 动漫, 游泳], job =Job{jobName='模特', jobAge=2}}
|
3.3 使用@PropertySource
@PropertySource
: 加载指定文件,
把上面有关Person
的配置信息,单独移到一个文件;这样以来就能和框架常用配置区分开。
3.3.1 提取到单独文件
新建文件: src/main/resources/person.properties
person.name=王麻子
person.age=21
person.is-boy=true
person.other.home=湖南 person.other.phone=1611090000000
person.likes=赌博,泡妞
person.birthday=1992/05/17
person.job.jobName=无业游民 person.job.jobAge=0
|
3.3.2 修改Person类
package com.hui.configfile.bean;
import lombok.Data; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.PropertySource; import org.springframework.stereotype.Component;
import java.util.Date; import java.util.List; import java.util.Map; @Data @Component
@PropertySource(value = "classpath:person.properties") @ConfigurationProperties(prefix = "person") public class Person { private String name; private Integer age; private Boolean isBoy; private Date birthday; private Map<String,Object> other; private List<String> likes; private Job job;
@Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + ", birthday=" + birthday + ", isBoy=" + isBoy + ", other=" + other.toString() + ", likes=" + likes.toString() + ", job =" + job.toString() + '}'; } }
|
3.3.3 编写测试代码
package com.hui.configfile;
import com.hui.configfile.bean.Person; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest public class testPerson { @Autowired private Person person;
@Test void run(){ System.out.println(person); } }
|
4.@Value
使用
4.1 使用方式
@Value
的常见的使用方式如下:
- 注入普通字符串
- 注入配置属性
- 注入系统变量
- 使用SpEL表达式
- 注入其他bean的属性
- 注入文件资源
- 注入网页资源
具体使用代码如下:
TestValue.java
: 用于演示 :注入其他bean的属性
package com.hui.configfile.bean;
import org.springframework.stereotype.Component;
@Component public class TestValue { public String desc = "我是TestValue的属性:desc"; }
|
ConfigFileApplicationTests.java
: 测试代码
package com.hui.configfile;
import com.hui.configfile.bean.TestValue; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.core.io.Resource;
import java.io.IOException;
@SpringBootTest class ConfigFileApplicationTests { @Value("${person.name}") private String name;
@Value("#{systemEnvironment['os.name']}") private String osName;
@Value("#{T(java.lang.Math).random()}") private double random;
@Autowired private TestValue testValue; @Value("#{testValue.desc}") private String beanProperty;
@Value("classpath:test.txt") private Resource localFile;
@Value("http://www.baidu.com") private Resource urlWeb;
@Test void run() throws IOException { System.out.println("读取配置中的基本类型-->" + name); System.out.println("读取系统变量-->" + osName); System.out.println("使用SpEL表达式-->" + random); System.out.println("注入其他bean的属性-->" + beanProperty); byte[] content = new byte[(int) localFile.contentLength()]; int read = localFile.getInputStream().read(content); String fileContent = new String(content); System.out.println("读取文件内容-->" + fileContent);
byte[] webContent = new byte[(int) urlWeb.contentLength()]; int read1 = urlWeb.getInputStream().read(webContent); String webString = new String(webContent); System.out.println("读取网页内容-->" + webString); } }
|
输出:
注入普通字符串-->哇哈哈哈哈哈 注入配置属性-->王麻子 注入系统变量-->null 使用SpEL表达式-->0.8653261032458481 注入其他bean的属性-->我是TestValue的属性:desc 注入文件资源-->JAVA,PHP,GO,C,PYTHON
注入网页资源--><!DOCTYPE html> <!--STATUS OK--><html> <head><meta http-equiv=content-type content=text/html;charset=utf-8><meta http-equiv=X-UA-Compatible content=IE=Edge><meta content=always name=referrer><link rel=stylesheet type=text/css href=http://s1.bdstatic.com/r/www/cache/bdorz/baidu.min.css><title>百度一下,你就知道</title></head> <body link=
|
4.2 ${}
和 #{}
区别
@Value
的值有两类:
5. @Value和@ConfigurationProperties区别
5.1 取值比较
@Value获取值和@ConfigurationProperties获取值比较
|
@ConfigurationProperties |
@Value |
功能 |
批量注入配置文件中的属性 |
一个个指定 |
松散绑定(松散语法) |
支持 |
不支持 |
SpEL |
不支持 |
支持 |
JSR303数据校验 |
支持 |
不支持 |
复杂类型封装 |
支持 |
不支持 |
5.2 使用场景
不管配置文件是application.yml
还是application.properties
,它们都能获取到配置中的值;
- 当我们只是需要获取配置文件中的某项值时,建议使用:
@Value
;
- 当我们专门编写了一个javaBean来和配置文件进行映射时,建议使用:
@ConfigurationPropertie
;