1.介绍
Elasticsearch(ES)
是一个基于Lucene
构建的开源、分布式、RESTful
接口的全文搜索引擎。Elasticsearch
还是一个分布式文档数据库,其中每个字段均可被索引,而且每个字段的数据均可被搜索,ES
能够横向扩展至数以百计的服务器存储以及处理PB级的数据。可以在极短的时间内存储、搜索和分析大量的数据。通常作为具有复杂搜索场景情况下的核心发动机。根据DB-Engines
的排名显示,Elasticsearch
是最受欢迎的企业搜索引擎。
1.1 集成流程
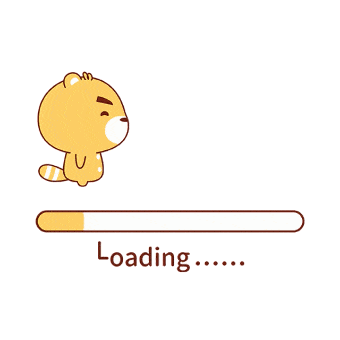
2.安装
这里使用olivere/elastic
,@注意: 下载包的版本需要和ES版本相同,如我们这里使用的ES是7.13.3的版本,那么我们就需要下载olivere/elastic/v7
。
go get github.com/olivere/elastic/v7
|
3. 配置
3.1 编辑主配置文件
文件位置:./config.yaml
elastic: enable: true url: http://127.0.0.1:9200 sniff: false healthcheckInterval: 5s logPre: ES-
|
3.2 新增配置结构体
文件位置: ./config/elastic.go
type elasticConfig struct { Url string `yaml:"url"` Sniff bool `yaml:"sniff"` HealthCheckInterval time.Duration `yaml:"healthCheckInterval"` LogPre string `yaml:"logPre"` commonConfig }
|
3.3 嵌入主配置
编辑文件:./config/app.go
type ServerConfig struct { ... Elastic elastic `yaml:"elastic"` }
|
3.4 定义全局变量
编辑文件:./global/global.go
var ( ... GvaElastic *elastic.Client )
|
4. 集成代码
4.1 集成入口
编辑文件:./main.go
func init() { ... initialize.InitES() } func main() { ... }
|
4.2 创建客户端
新建文件:./initialize/elastic.go
func InitES() { elasticConfig := global.GvaConfig.Elastic if elasticConfig.Enable { fmt.Printf("elasticConfig: %v\n", elasticConfig) client, err := elastic.NewClient( elastic.SetURL(elasticConfig.Url), elastic.SetSniff(elasticConfig.Sniff), elastic.SetHealthcheckInterval(elasticConfig.HealthCheckInterval), elastic.SetErrorLog(global.GvaLogger), elastic.SetInfoLog(global.GvaLogger), ) if err != nil { panic("创建ES客户端错误:" + err.Error()) } global.GvaElastic = client } }
|
5. 测试使用
5.1 创建控制器
新建文件:./api/v1/es_api.go
const indexName = "go-test"
func SearchById(ctx *gin.Context) { id,_ := ctx.GetQuery("id") res, err := global.GvaElastic.Get().Index(indexName).Id(id).Do(ctx) if err != nil { response.Error(ctx, fmt.Sprintf("查询失败:%s",err)) return } response.OkWithData(ctx,res.Source) }
|
5.2 注册路由
新建文件:./api/v1/es_router.go
func InitESRouter(engine *gin.Engine) { esGroup := engine.Group("es") { esGroup.GET("searchById", v1.SearchById) } }
|
5.3 请求返回
curl -XGET http://127.0.0.1:8080/es/searchById?id=5
{"code":0,"msg":"请求成功","data":{"name":"李四","age":22,"birth":"1992-04-25"}}
|